Form input commands
These commands are used to set values for form input and validate form inputs.
List of commands
Input commands
Command | Description |
---|---|
I.fill |
Fill a textual field |
I.select |
Select a dropdown option, checkbox, or radio button |
I.deselect |
Deselect a checkbox |
I.upload |
Upload a file |
Assertion / Validation commands
Command | Description |
---|---|
I.filled |
Assert that the form is filled with a specific value |
I.selected |
Assert that a dropdown option, checkbox, or radio button is selected |
I.deselected |
Assert that a dropdown option, checkbox, or radio button is NOT selected |
I.fill
Short form of
I.fillField
command
Fill a textual field.
This works for date picker fields that accept direct text input.
Usage
I.fill(field, value)
Parameters
Parameter | Type | Remarks |
---|---|---|
field | string | Keyword to identify the field. The associated label, aria-label, placeholder, adjacent text, and current value can be used to identify a field. CSS selectors can also be used, but is not recommended as it makes the tests harder to keep up to date. |
value | string | Value to fill into the field |
Example(s)
Fill a field
I.fill("Email", "[email protected]");
This command fills "[email protected]" into the "Email" field.
Clear a field
// fill with empty value
I.fill("Email", "");
// or
I.clear("Email");
This command clears the "Email" field.
I.filled
Asserts that the form is filled with a specific value
Usage
I.filled(field, value)
Parameters
Parameter | Type | Remarks |
---|---|---|
field | string | Keyword to identify the field. The associated label, placeholder, adjacent text, and name can be used to identify a field. CSS selectors can also be used, but is not recommended as it makes the tests harder to keep up to date. |
value | string | Value that the field is expected to have |
I.select
Select a dropdown, checkbox, or radio button.
This -ONLY- works on native HTML5 elements.
For custom elements, useI.click
as a fallback.
Usage
Select an option
:
I.select(option)
Select an option
in a list
:
I.select(list, option)
Parameters
Parameter | Type | Remarks |
---|---|---|
list | string | (Optional) Keyword to identify the options list |
option | string | Keyword to identify the option to select |
Example(s)
Select a dropdown
Here, we will use this "Number" dropdown list for illustration.
Specify the option
;
I.select("Two");
Or, specify the list
and the option
:
I.select("Number", "Two");
This selects the option "Two".
Select a checkbox
Here, we will use this check box for illustration.
I.select("I agree to the terms and conditions");
This selects the checkbox "I agree to the terms and conditions".
Select a check box in a checkbox list
Here, we will use this "Color" checkbox list for illustration.
Specify the option
;
I.select("Red");
Or, specify the list
and the option
:
I.select("Color", "Red");
This selects the option "Red".
Select a radio button
Here, we will use this "Animal" radio button list for illustration.
Specify the option
;
I.select("Cat");
Or, specify the list
and the option
:
I.select("Animal", "Cat");
This selects the option "Cat".
I.selected
Asserts that a dropdown option, checkbox, or radio button is selected
Usage
I.selected(option)
I.selected(list, option)
Parameters
Parameter | Type | Remarks |
---|---|---|
list | string | Keyword to identify the options list |
option | string | Keyword to identify the option to be selected |
I.deselect
Deselect a checkbox.
Usage
Deselect an option
:
I.deselect(option)
Deselect an option
in a list
:
I.deselect(list, option)
Parameters
Parameter | Type | Remarks |
---|---|---|
list (optional) | string | Keyword to identify the options list |
option | string | Keyword to identify the option to deselect |
I.deselected
Asserts that a dropdown option, checkbox, or radio button is NOT selected
Usage
I.deselected(option)
I.deselected(list, option)
Parameters
Parameter | Type | Remarks |
---|---|---|
list | string | Keyword to identify the options list |
option | string | Keyword to identify the option that expected to be NOT selected |
I.upload
Upload a file.
Usage
I.upload(field, pathToFile)
Parameters
Parameter | Type | Remarks |
---|---|---|
field | string | Keyword to identify the file upload field |
pathToFile | string | Path to the file to upload. Currently, only absolute paths from the project root are supported. |
Example
First, you need to add the file to be used for testing file upload to the UI-licious test project.
Click on the "plus" button in the workspace pane to open the "Add file" dialog.
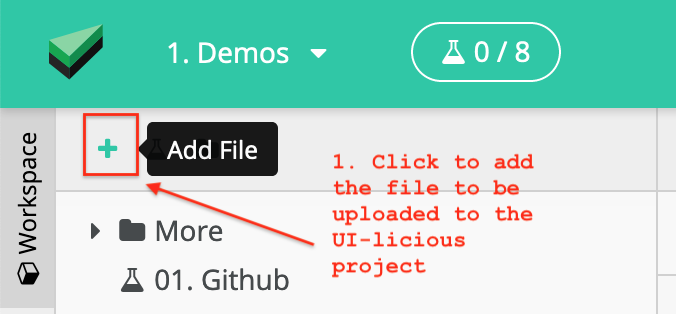
Select the "Upload file" menu, and upload the file to the project.
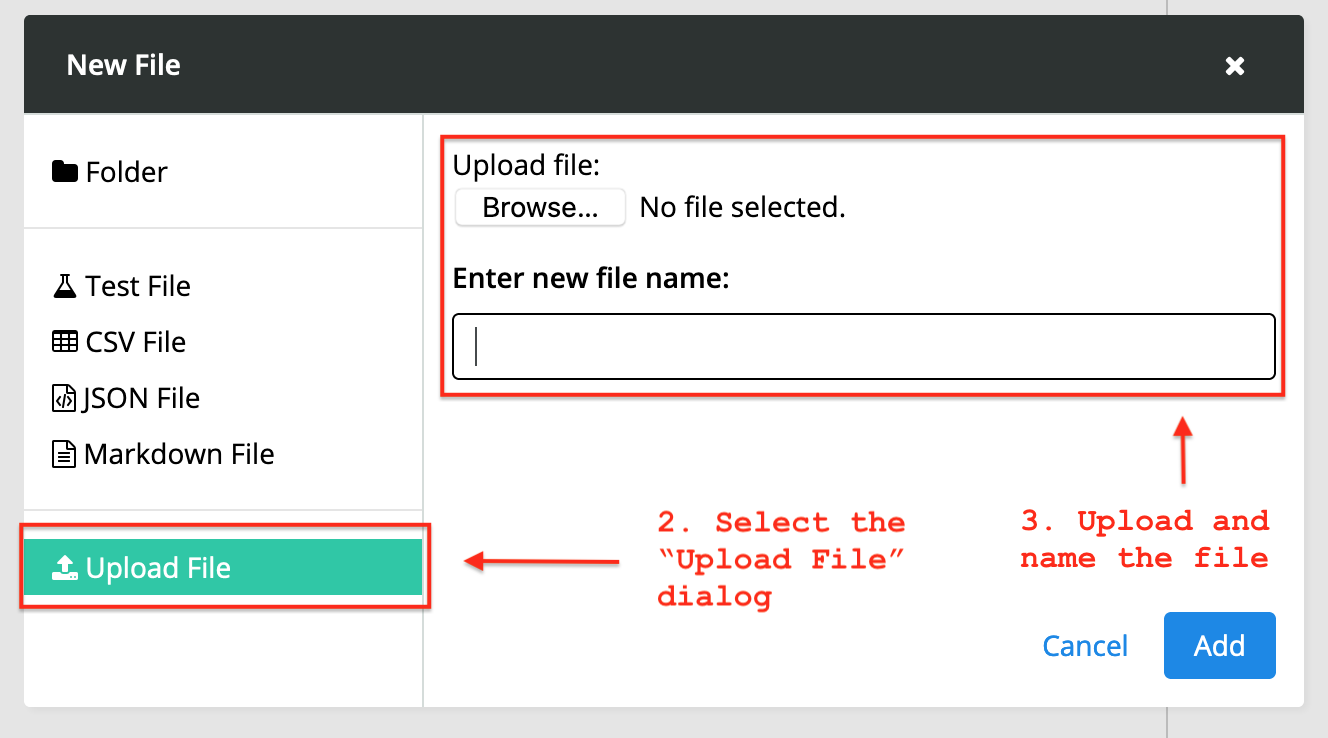
Finally, specify the absolute or relative path to the file in the I.upload
command.
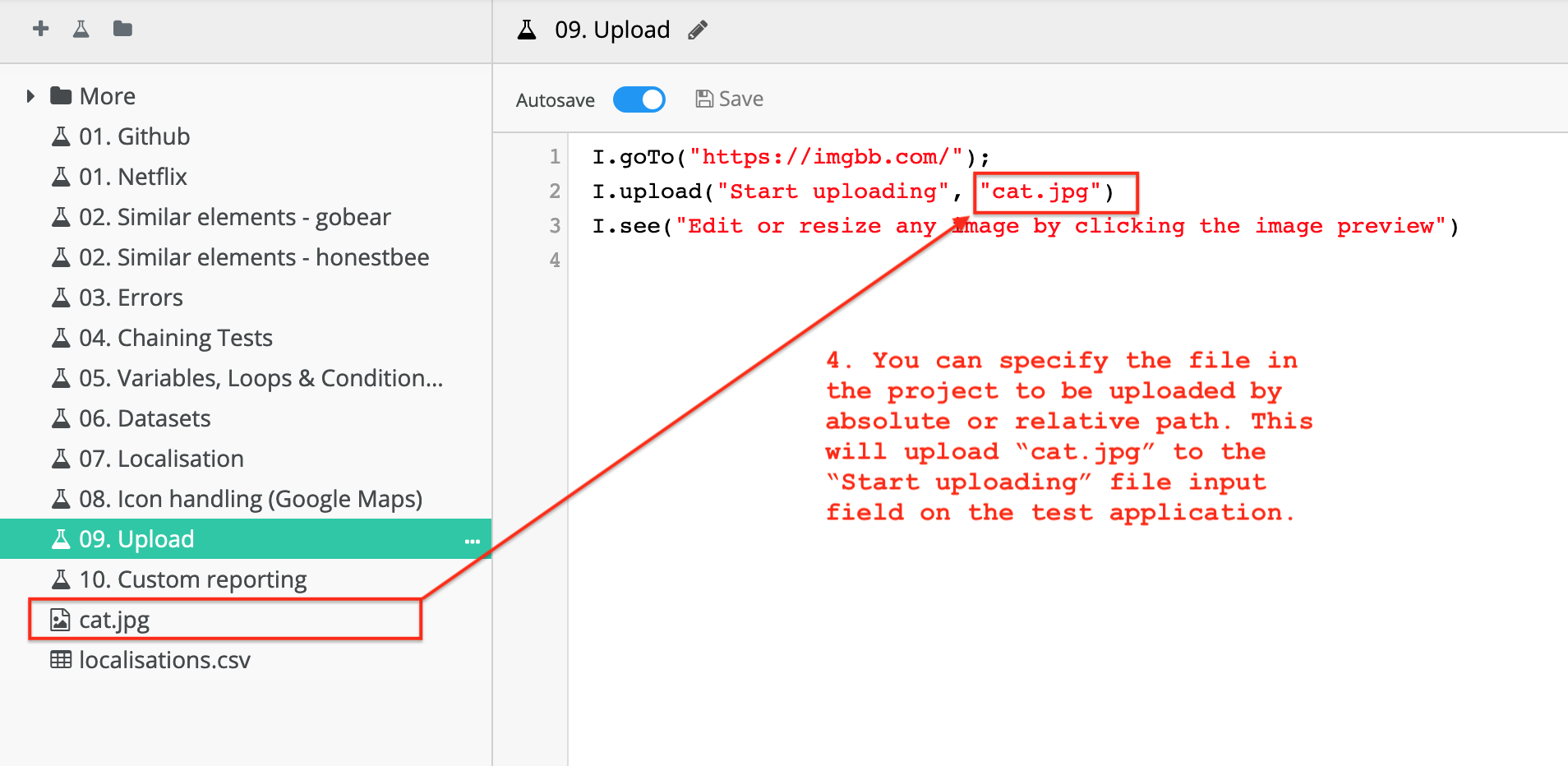
This will upload "cat.jpg" to the "Start uploading" file input field in our test application.